In the realm of Ruby programming, a thread embodies the capability to concurrently execute multiple tasks, thereby enhancing the efficiency and versatility of your programs. Harnessing threads empowers your Ruby scripts to engage in simultaneous activities, culminating in the creation of a multi-threaded environment that accelerates task completion.
Threads, essentially acting as diligent workers or individual units of execution within a program, are pivotal in facilitating this parallelism. Each process inherently encompasses at least one thread, with the flexibility to spawn additional threads as required, thereby accommodating varying workloads and operational demands.
Before delving into a concrete code demonstration, it’s imperative to grasp the distinction between CPU-bound and I/O-bound applications, as this understanding lays the groundwork for effective thread management and utilization.
Exploring I/O Bound Applications
In the realm of application development, I/O bound applications stand out as fascinating entities that rely heavily on external resources. These applications, while adept at executing tasks, often find themselves in a waiting game for essential elements such as API responses, database query results, or disk reads. However, they possess a unique characteristic that allows them to manage this waiting period effectively without halting entirely: the prowess of threading.
Understanding Threading Dynamics
Threading serves as a cornerstone in the realm of I/O bound applications. By leveraging threads, these applications can efficiently handle waiting times for external resources without sacrificing productivity. Here’s how threading dynamics come into play:
- Concurrency: Threading enables multiple tasks to execute concurrently, allowing an I/O bound application to perform other operations while waiting for external resources;
- Resource Utilization: Threads optimize resource utilization by allowing the application to switch between tasks seamlessly, ensuring that no computing power is wasted during waiting periods;
- Parallelism: Through threading, an I/O bound application can achieve parallelism, enabling it to make progress on multiple fronts simultaneously.
Illuminating Examples
To shed light on the concept of I/O bound applications, let’s consider the quintessential example of a web crawler. This digital arachnid scours the internet, fetching data from various servers. However, with each request, it must patiently await the server’s response before proceeding. Without threading, this waiting time could significantly hinder its efficiency.
Optimizing Performance with Threading
Now, imagine the transformative power of threading in enhancing the performance of our web crawler. By employing threads, we can revolutionize its operational efficiency:
- Concurrent Requests: With threading, the web crawler can initiate multiple requests simultaneously, reducing the overall waiting time for server responses;
- Asynchronous Processing: Threads enable the crawler to handle responses as they arrive, allowing it to continue its data-fetching mission without unnecessary delays;
- Enhanced Throughput: By making multiple requests concurrently, threading amplifies the crawler’s throughput, enabling it to fetch pages at a significantly faster rate.
Practical Implementation Strategies
Harnessing the potential of threading requires strategic implementation. Here are some practical strategies to maximize the benefits of threading in I/O bound applications:
- Thread Pooling: Implement a thread pool mechanism to manage and recycle threads efficiently, preventing resource wastage;
- Optimal Thread Count: Determine the ideal number of threads based on system specifications and workload characteristics to achieve optimal performance without overwhelming system resources;
- Error Handling: Implement robust error handling mechanisms to gracefully manage exceptions and failures that may arise during threaded operations;
- Monitoring and Tuning: Continuously monitor application performance and fine-tune threading strategies to adapt to evolving workloads and resource dynamics.
Unlocking the Power of Ruby Threads
In the world of Ruby programming, threading is a powerful tool that enables concurrent execution of tasks, allowing for improved performance and responsiveness in your applications. Understanding how to create and manage threads effectively can greatly enhance the functionality and efficiency of your Ruby programs. Let’s delve into the intricacies of creating Ruby threads and handling exceptions.
Creating Ruby Threads: A Step-by-Step Guide
Creating a new Ruby thread is a straightforward process, primarily done by invoking the Thread.new method. Here’s a step-by-step guide to get you started:
Call Thread.new Method: Begin by invoking Thread.new and passing in a block containing the code that you want the thread to execute. This block encapsulates the instructions that the thread will carry out asynchronously.
Example:
Thread.new { puts “hello from thread” }
- Ensure Proper Execution: It’s crucial to ensure that the code block provided to the Thread.new method contains the necessary instructions for the thread’s execution. Without a block or proper code execution within the block, the thread may not function as intended;
- Handling Output: When creating threads, especially in scenarios where output is expected, ensure that the thread’s execution produces the desired output. Debugging any issues related to output can streamline the development process;
- Managing Thread Completion: By default, Ruby doesn’t wait for threads to finish execution, potentially leading to unexpected behavior. To address this, use the join method to synchronize thread execution with the main program flow.
Example:
t = Thread.new { puts 10**10 }
puts "hello"
t.join
Utilizing Multiple Threads: To harness the full potential of concurrency, you can create and manage multiple threads efficiently. Store thread objects in an array and utilize the join method to synchronize their execution.
Example:
threads = []
10.times {
threads << Thread.new { puts 1 }
}
threads.each(&:join)
Threads and Exception Handling: Ensuring Robustness
Exception handling is essential when working with threads to ensure the robustness and stability of your Ruby applications. Here’s how you can handle exceptions effectively:
- Understanding Thread Behavior: In Ruby, if an exception occurs within a thread, it may terminate silently without disrupting the main program flow or providing error messages;
- Debugging Considerations: During development and debugging phases, it’s beneficial to have threads halt execution upon encountering exceptions. This facilitates error identification and troubleshooting;
- Enabling Exception Handling: To configure threads to raise exceptions and halt execution upon encountering errors, set the abort_on_exception flag to true. It’s advisable to set this flag before creating any threads in your program.
Example:
Thread.abort_on_exception = true
Optimizing Resource Usage with Thread Pools
Processing a vast number of items concurrently without careful management can lead to resource depletion and system overload. Imagine launching hundreds of connections against a server simultaneously—it’s a recipe for disaster. Fortunately, there are smarter ways to handle such tasks, and one effective solution is utilizing thread pools.
What are Thread Pools?
Thread pools serve as a mechanism to regulate the number of active threads operating at any given time. Instead of creating a new thread for each task, which could overwhelm system resources, thread pools efficiently manage a limited set of threads to execute multiple tasks in a controlled manner.
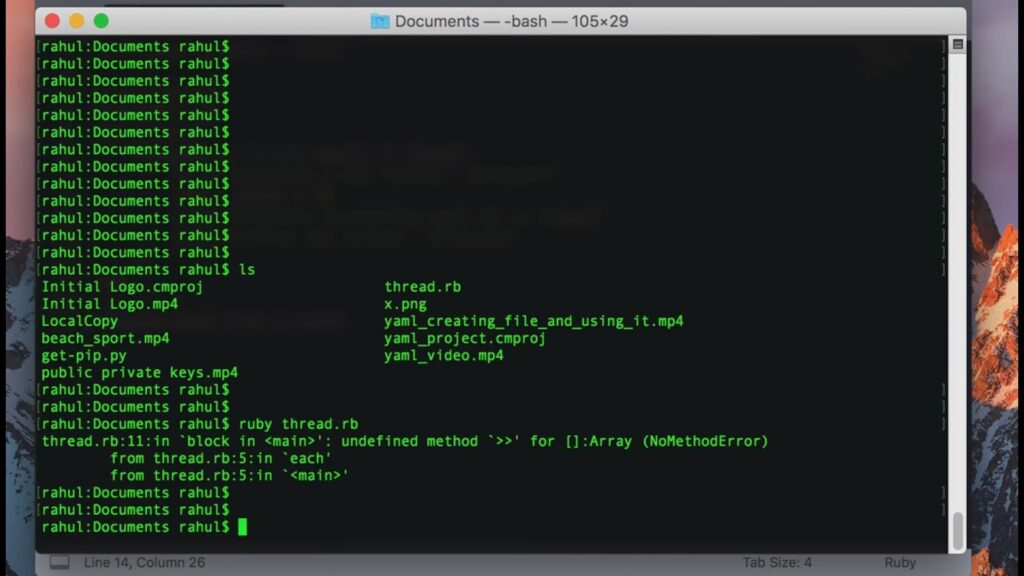
Advantages of Thread Pools:
- Resource Management: Thread pools prevent excessive consumption of system resources by limiting the number of active threads;
- Enhanced Performance: By reusing existing threads, thread pools reduce the overhead of thread creation, leading to improved performance;
- Scalability: Thread pools facilitate the management of concurrent tasks, allowing applications to scale efficiently without compromising stability.
Implementing Thread Pools with Celluloid Gem
While building a custom thread pool is possible, leveraging existing solutions like the Celluloid gem streamlines the process. Let’s delve into how you can utilize Celluloid to manage thread pools effectively.
require 'celluloid'
class Worker
include Celluloid
def process_page(url)
puts url
# Perform processing tasks here
end
end
pages_to_crawl = %w(index about contact products ...)
worker_pool = Worker.pool(size: 5)
# Submit tasks to the thread pool
pages_to_crawl.each { |page| worker_pool.process_page(page) }
In this example, only five threads are active at any time, ensuring optimal resource utilization and preventing system overload. As threads complete their tasks, they automatically pick up the next item in the queue, enhancing efficiency.
Addressing Concurrent Code Challenges
While utilizing thread pools offers numerous benefits, it’s essential to understand and mitigate potential challenges associated with concurrent code, such as race conditions and deadlocks.
Common Challenges:
- Race Conditions: Occur when multiple threads access shared resources simultaneously, leading to unpredictable behavior and data corruption;
- Deadlocks: Arise when threads compete for exclusive access to resources, resulting in a situation where each thread is waiting for the other to release a lock, causing a standstill in execution.
Best Practices for Handling Concurrent Code:
- Avoid Raw Threads: Utilize established libraries or gems that abstract low-level thread management to minimize the risk of concurrency issues;
- Implement Locking Mechanisms: Use synchronization primitives like mutexes to control access to shared resources and prevent race conditions;
- Thorough Testing: Rigorously test concurrent code to identify and address potential issues before deployment.
Conclusion
In conclusion, threads in Ruby serve as indispensable tools for facilitating concurrent execution and enhancing program performance. By embracing threads, developers unlock the potential for multi-threaded Ruby programs, enabling faster task execution and improved efficiency. Understanding the nuances between CPU-bound and I/O-bound applications is crucial for effective thread management and optimizing program behavior. Embracing threads empowers developers to leverage the full potential of Ruby for creating robust and responsive applications.