Should you seek to execute an external command in Ruby, such as employing wkhtmltopdf for the conversion of an HTML document into a PDF format, there exist several Ruby techniques at your disposal. The outcome of your endeavor may vary significantly based on the specific method employed. Let us embark on an exploration of these diverse methodologies together!
The Methodology of Ruby System Commands
The system method in Ruby offers a straightforward approach to executing external commands. This accessible feature allows you to interact and engage with the operating system straightly and promptly.
Executing a system command in Ruby looks something like this:
system(“ls”)
In this instance, ls is a Linux command that lists all files and directories in the current directory. When executed, the Ruby system method prints out the result of the command in real-time directly to the console.
One key feature to note is that the system method temporarily halts the execution of the Ruby program until the external command has completely run. For example:
system(“sleep 2”)
This will pause the entire program for 2 seconds, emphasizing the linear execution flow of system.
However, it’s not always required to pause the Ruby program. Advanced techniques allow commands to run concurrently or in the background, which we will explore in the later sections of this tutorial.
The Ruby system method can yield three potential return values:
- true: The command was successful;
- false: The command ran, but returned an error code;
- nil: The command execution failed completely, usually due to the command not being found.
To further understand the reason behind a command failure, the global variable $? can be used to fetch the exit status code of the most recently executed external command. It provides greater insight into any errors or issues that might have occurred during the command’s execution.
Understanding Shell Expansion: The Core of Ruby’s System Method
Shell Expansion is a robust feature automatically incorporated when executing an external command with Ruby’s system method. It’s at the heart of many command line operations and it’s a fundamental topic to grasp for every Ruby developer.
The process of Shell Expansion sounds complicated but it’s easier to understand with examples. When a shell (bash, zsh, or sh) encounters special characters like *, ?, or [], it expands them into a list of files.
Consider this example:
system("echo *")
In this command, the asterisk (*) stands for all files and folders in the current directory. This means when the command is executed, it will print every file and folder found in the present directory. It can be particularly useful when you need to review the contents of a directory quickly and efficiently.
A crucial point to remember is that the * is replaced with the actual list of files before the command gets executed. This process is known as Shell Expansion.
However, there may be instances where you want these special characters to retain their original meaning and not get expanded by the shell. In such scenarios, you pass these special characters as the second argument of the system method.
Here is how to do this:
system("echo", "*")
In this case, the output will be the single asterisk (*) character and not the files in the directory. It’s a fundamental difference that adds another layer of control and flexibility to your command execution. This method becomes highly useful when you need to work with special characters in their literal form.
In summary:
- Shell Expansion is a core concept in the functioning of the Ruby system method;
- Special characters such as *, ?, and [] trigger Shell Expansion;
- To prevent Shell Expansion, pass special characters as the second argument of system.
By understanding and leveraging Shell Expansion, you can run more complex commands, open up considerable automation possibilities, and thus elevate your overall Ruby programming.
Practical Usage of Environment Variables in Ruby’s System Method
Environment Variables in Ruby provide a powerful way to feed configuration values into your external commands. They offer a brilliant solution when there are values to be passed to your external commands without altering the present environment of your Ruby program.
Let’s delve into an example to see Environment Variables in action:
system({"myRubyApp" => "awesome"}, "ruby", "-e p ENV['myRubyApp']")
# "awesome"
In this command, the Environment Variable myRubyApp is set to awesome. This value can then be accessed through the ENV hash in Ruby.
Do note that this doesn’t impact your application’s current environment. The Environment Variable only persists through the execution of the specific command.
Availing the Power of %x and Kernel#“ in Ruby
In Ruby, %x and Kernel#“ (backtick method) are unique ways to capture the output of an external command. Instead of merely running the command and displaying the output, these methods enable you to store the output.
Consider the following examples:
`ls`
And:
%x|ls|
Both examples will return a string with the output of the ls command. This output can then be manipulated or used within your Ruby code as per your requirements, adding another level of interactivity to your Ruby programs.
However, remember that your Ruby program will wait for the external command to finish before it proceeds, unless the command is run in a separate thread. This behavior ensures that your program has the complete output before continuing with its next steps.
To recap:
- Environment Variables can be passed through a hash to the system method;
- %x and Kernel#“ are used to capture and use the output from an external command;
- The default behavior is to pause your Ruby program until the external command has finished, unless run in a separate thread.
Leveraging Fork and Exec to Run External Commands Concurrently
In Ruby, Fork and Exec provide a powerful mechanism to run multiple processes in parallel, boosting program efficiency. By making clever use of these commands, you can significantly enhance the concurrency and multitasking capabilities of your Ruby applications.
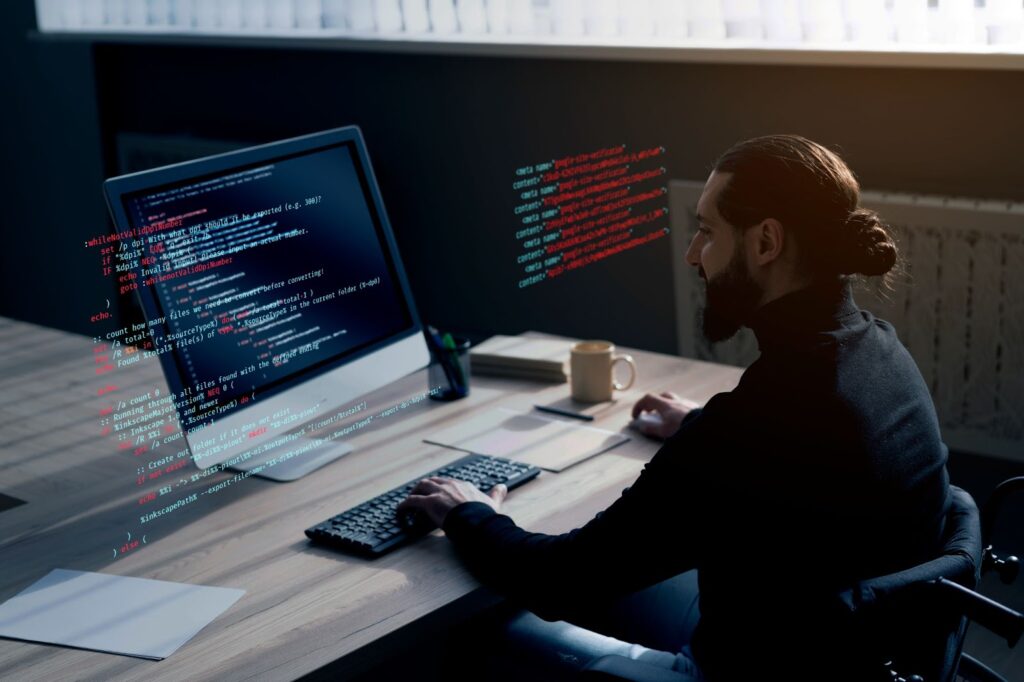
Fork is a system call in Unix-based systems that creates a new process by duplicating the existing one. The newly created process, called the child, is an exact copy of the parent process. However, the child process may be replaced later using the Exec function.
Unfortunately, the Fork method is not available on Windows systems.
The Exec function in the meanwhile replaces the current process with a new one. The replacement process starts from its main function as if it is a brand new process.
Look at this code below:
fork { exec("ls") }
The line of code has the effect of producing a duplicate process, then replacing that duplicate with the ls process. An important point to remember is that this command will be running independently, without blocking your Ruby application, unlike the system method or %x. This command is executed in a new process.
However, using Exec without Fork can immediately end your Ruby program. Exec replaces the current process entirely, and once replaced, the original process ceases to exist. Therefore, the execution of your Ruby program halts abruptly.
Key takeaways:
- Fork and Exec are powerful commands to run external commands concurrently in Ruby;
- While Fork creates a duplicate process, Exec replaces an existing process with a new one;
- The usage of Exec without Fork can terminate your Ruby application instantaneously;
- The execution of Fork and Exec does not block the Ruby application.
Leveraging these commands into your everyday Ruby programming will result in improved efficiency and concurrency in your programs.
Mastering IO.popen: Two-way Communication with External Programs
In Ruby, IO.popen is a method that opens a process for both reading and writing. It is essentially designed for when you need more interactivity and control with an external process, and when you require two-way communication.
The IO.popen method can launch an external process and allow for the exchange of inputs and outputs directly in your Ruby code.
For instance, consider this example:
interactive_ruby = IO.popen("irb", "r+")
interactive_ruby.write "puts 123 + 1\n"
3.times { puts interactive_ruby.gets }
interactive_ruby.write "exit\n"
In Ruby programming, the IO.popen method serves as a powerful tool for seamlessly integrating external processes into your applications. By leveraging IO.popen, you can establish a direct channel to interactive shells like Interactive Ruby (irb), enabling bidirectional communication with inputs and outputs right within your Ruby program.
Harnessing the Power of IO.popen
When utilizing IO.popen, you’re essentially launching an external shell, such as irb, and facilitating a fluid exchange of data. Here’s a breakdown of its functionality and benefits:
- Interactive Communication:
- IO.popen establishes a bridge between your Ruby code and external programs, enabling real-time interaction;
- Inputs can be passed to the external shell, and outputs can be seamlessly retrieved within your Ruby application.
- Non-blocking Execution:
- Despite executing commands in an external shell, your Ruby program remains unblocked, ensuring smooth execution without hindering performance;
- Each command runs within its own process space, preserving the integrity and efficiency of your Ruby application.
- Enhanced Control with popen3:
- Ruby’s standard library features a variant of IO.popen known as popen3;
- Unlike the traditional popen method, popen3 segregates standard output and error messages into distinct IO objects, enhancing clarity and manageability.
- Two-way Communication:
- IO.popen facilitates two-way communication, allowing your Ruby application to both send commands and receive responses from external processes;
- This seamless exchange of data empowers you to orchestrate complex workflows and integrations effortlessly.
- Improved Error Handling:
- With popen3, error messages are isolated from standard output, simplifying error detection and troubleshooting;
- This distinction between output and error streams enhances the robustness and reliability of your Ruby applications.
Conclusion
In conclusion, navigating the realm of executing external commands in Ruby offers a spectrum of methodologies, each yielding distinct outcomes. By understanding and employing these varied techniques, you can wield greater control and efficiency in your Ruby projects.