If you’re a Ruby programmer, you’ve probably heard of structs and OpenStructs. But do you know how to use them effectively? In this tutorial, we’ll cover everything you need to know about structs and OpenStructs in Ruby, from what they are to how to use them in your code. So let’s dive in!
How to Create Structs in Ruby?
A struct is a built-in Ruby class that allows you to create new classes which produce value objects. These value objects are used to store related attributes together. For example, if you have a Point with two coordinates (x and y), you can represent this data in different ways, such as an array [10, 20], a hash { x: 10, y: 20 }, or an object Point.new(10, 20). However, if you have multiple Points, it’s best practice to use the object approach. But creating a whole class just to store two values together can be cumbersome. This is where structs come in handy.
To create a struct in Ruby, you can use the Struct.new method and pass in a list of symbols that will become the instance variables of the class. These variables will have accessor methods defined by default for both reading and writing. Let’s look at an example:
Person = Struct.new(:name, :age, :gender)
Now, you can create objects of this struct like this:
john = Person.new("John", 30, "M")
david = Person.new("David", 25, "M")
Alternative Way
You may come across another way of creating structs in the wild, which looks like this:
class Person < Struct.new(:name, :age, :gender)
end
While this may work, it’s not recommended. It creates a subclass of the Struct class and can lead to unexpected behavior. Stick with using the Struct.new method for creating structs.
Big Benefit
One of the biggest benefits of using structs is that you can compare them directly based on their attributes. This means you can easily sort or filter an array of structs based on a specific attribute without having to write any custom comparison methods.
How to Use Ruby Structs?
Now that you know how to create a struct in Ruby, let’s look at some ways you can use them in your code.
WARNING: Structs Can Be Tricky
While structs may seem like a simple and convenient way to store related attributes together, they can be tricky to use if you’re not careful. One thing to keep in mind is that structs are mutable, meaning their values can be changed after they’ve been created. This can lead to unexpected results if you’re not aware of it. Let’s take a look at an example:
Person = Struct.new(:name, :age)
john = Person.new("John", 30)
david = Person.new("David", 25)
people = [john, david]
# Change John's age
john.age = 35
# Now both john and people[0] have an age of 35
puts john.age
# Output: 35
puts people[0].age
# Output: 35
As you can see, changing the value of john also changes the value of people[0]. This is because structs are passed by reference, not by value. To avoid this issue, you can use the freeze method to make your structs immutable, but this can also lead to other problems if you try to modify them later on.
Another Weird Thing
Another weird thing about structs is that they don’t inherit from the Object class like other Ruby classes do. This means they don’t have access to methods like to_s or inspect. However, you can still define these methods in your struct to customize how it’s represented as a string or when using puts or p on it.
Named Parameters in Ruby 2.5
Starting with Ruby 2.5, you can use named parameters when creating structs. This allows you to specify default values for your struct’s attributes and also makes your code more readable. Let’s take a look at an example:
Person = Struct.new(:name, :age, :gender, keyword_init: true)
john = Person.new(name: “John”, age: 30, gender: “M”)
david = Person.new(name: “David”, age: 25, gender: “M”)
As you can see, we no longer need to pass in the attributes in a specific order, and we can also omit any attributes that have default values. This makes it easier to create structs with many attributes without having to remember their order.
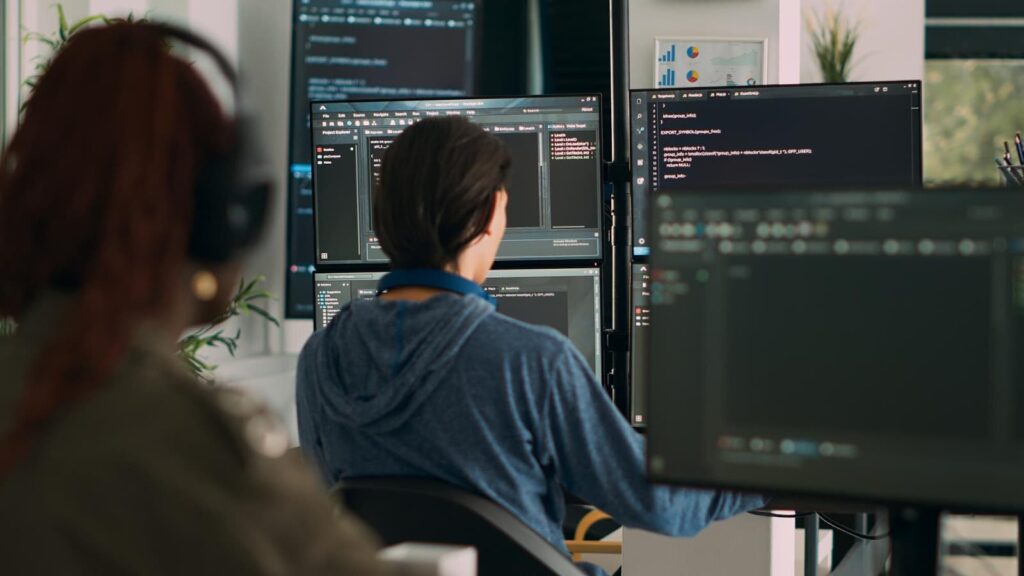
Struct vs OpenStruct
Now that we’ve covered structs, let’s talk about OpenStructs. An OpenStruct is another built-in Ruby class that allows you to create objects with arbitrary attributes. The main difference between a struct and an OpenStruct is that an OpenStruct’s attributes are not defined beforehand. Instead, you can add new attributes to an OpenStruct object at any time. Let’s look at an example:
require 'ostruct'
person = OpenStruct.new
person.name = "John"
person.age = 30
person.gender = "M"
puts person.name
# Output: John
puts person.age
# Output: 30
puts person.gender
# Output: M
As you can see, we can add new attributes to the person object after it’s been created. This can be useful in certain situations, but it’s important to note that OpenStructs are slower than structs because they use method_missing to handle attribute assignments and retrievals.
So when should you use a struct vs an OpenStruct? It depends on your specific needs. If you know all the attributes you’ll need beforehand and don’t need to add new ones dynamically, then a struct is a better choice. However, if you need the flexibility of adding new attributes at runtime, then an OpenStruct may be a better option.
Watch Video Tutorial
If you prefer visual learning, check out this video tutorial on how to use structs and OpenStructs in Ruby:
Conclusion
In this tutorial, we covered everything you need to know about using structs and OpenStructs in Ruby. We learned how to create structs, their benefits and potential pitfalls, and how they differ from OpenStructs. We also discussed the use of named parameters in Ruby 2.5 and when to use a struct vs an OpenStruct. With this knowledge, you can now confidently use structs and OpenStructs in your Ruby code.