Welcome to a comprehensive guide on generating random numbers and strings in Ruby. In this guide, we delve into the various ways Ruby enables developers to generate random numbers and strings, exploring the practical uses for these features and showcasing examples along the way.
Random numbers can serve various purposes, from choosing a random element from an array to selecting a winner from a list, or even simulating dice rolls. With Ruby, such scenarios become really simple to implement.
Mastering Random Number Generation in Ruby
Random numbers are incredibly useful for a variety of tasks, such as selecting a random item from an array, choosing a contest winner, or simulating dice rolls.
Ruby offers multiple methods for generating random numbers, each with its unique characteristics.
For instance, the rand method is versatile, allowing for three distinct uses:
- By default, rand returns a float between 0 and 1 (e.g., 0.4836732493);
- When given an integer argument (rand(10)), it generates a random integer within the 0 to that number range;
- With a range argument (rand(1..20)), it produces an integer within the specified range.
Ruby also provides other randomness generation techniques, including:
- The Array#shuffle method for rearranging array elements randomly;
- The Array#sample method for picking one or more random elements from an array;
- The SecureRandom class for generating secure random numbers and strings.
Let’s delve into some examples to illustrate these methods in action!
Generating Random Numbers
The code snippet from the image is:
[2] pry(main)> rand
=> 0.24710205493880377
When invoked without arguments, rand in Ruby generates floating-point numbers, such as 0.4836732493.
To specify a range, you can provide an argument to rand to produce a number from 0 up to (but not including) the given value. For instance:
rand(100)
# Output: 41
While Ruby’s random number generation is straightforward, sometimes you may require numbers within a specific range rather than starting from zero. Luckily, this can be easily achieved.
You can utilize a range to precisely define the desired range. For example:
rand(200..500)
# Output: 352
This will generate a random number between 200 and 499 (inclusive), providing the flexibility to tailor the generated numbers to your specific requirements.
Secure Random Number Generation in Ruby
In Ruby, the basic rand function might suffice for straightforward tasks within a basic application. However, when it comes to operations demanding a higher level of security — such as the creation of a password reset token — it’s imperative to opt for SecureRandom. This module is a robust component of the Ruby standard library, specifically designed for generating secure random numbers.
SecureRandom distinguishes itself by sourcing its randomness from system-specific, secure origins. On Unix-based systems, it leverages /dev/urandom for this purpose, while on Windows, it utilizes the CryptAcquireContext and CryptGenRandom APIs. This ensures that the random numbers generated are suitable for cryptographic applications.
Consider this demonstration of its usage:
require 'securerandom'
SecureRandom.random_number
> 0.232
This example illustrates the basic functionality, which mirrors that of rand, with the added capability of specifying a maximum value:
SecureRandom.random_number(100)
> 72
SecureRandom offers alternative output formats.
Utilizing hex output can produce a fixed-width hexadecimal string.
SecureRandom.hex
> "87694e9e5231abca6de39c58cdfbe307"
In Ruby 2.5, a new method was introduced to generate random alphanumeric strings.
SecureRandom.alphanumeric
> "PSNVXOeDpnFikJPC"
Methods for Selecting Random Elements from Collections
If you’re exploring ways to randomly select items from a collection, consider the following approach:
[5, 15, 30, 60].shuffle.first
> 30
However, Ruby offers a more efficient and direct method for this purpose, the sample method, which not only is faster but also specifically designed for random selection:
[5, 15, 30, 60].sample
> 5
The sample method is versatile and can also be applied to ranges. Below is an example that illustrates how to randomly generate a letter:
('a'..'z').to_a.sample
> b
You have the option to provide an integer argument to the “sample” function in order to obtain N distinct elements from the array.
[1,2,3].sample(2)
> [1, 2]
You can also provide a custom random generator as an argument if needed.
[1,2,3].sample(random: SecureRandom)
Generating Random Strings in Ruby
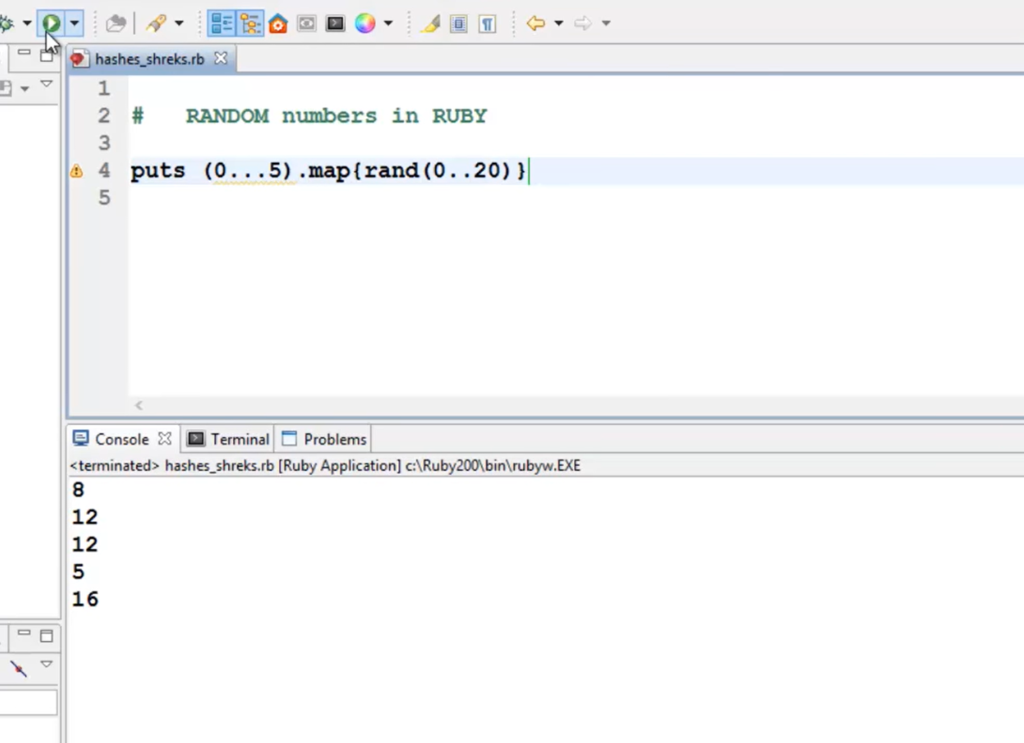
One of the most versatile applications of randomness in Ruby is the generation of random strings using a customized character set. Below is an example of the code to accomplish this:
def generate_code(number)
charset = Array('A'..'Z') + Array('a'..'z')
Array.new(number) { charset.sample }.join
end
puts generate_code(20)
Here are several steps involved in the code:
- Initially, we create our character set by defining ranges and converting them into arrays. Then, we utilize the capability of calling Array.new with a block. This allows us to initialize an array of size n with values generated by the block;
- The resulting strings from this code will have a format similar to: TufwGfXZskHlPcYrLNKg;
- Feel free to adjust the character set according to your requirements.
Random Number Generation Through Seed Initialization
To exert influence over the sequence of numbers produced by random number generation functions, such as rand, it’s possible to initialize the random number generator with a specific seed value.
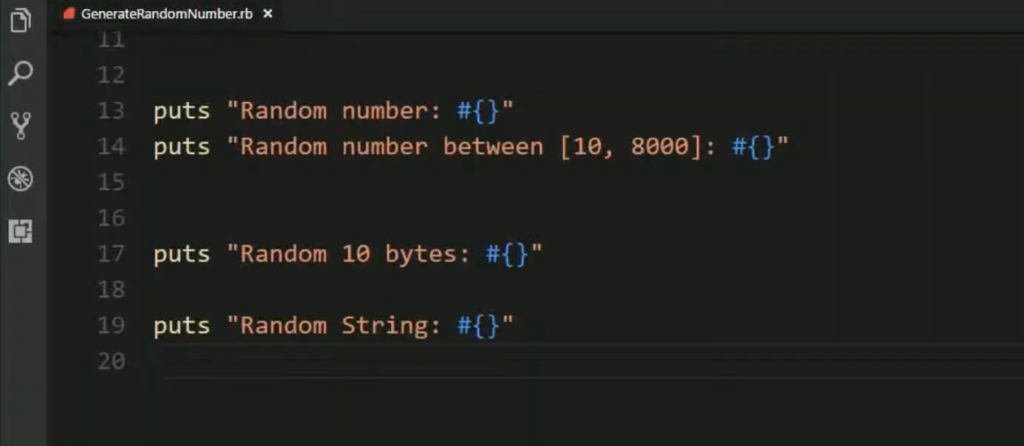
What exactly is a seed in this context?
- A seed serves as the initial value that kick-starts the creation of a sequence of random numbers. The entirety of the sequence is dependent on this initial seed value. This is why the selection of an appropriate seed is crucial for generating a high-quality sequence of random numbers;
- Programming languages like Ruby offer built-in mechanisms for seed management, ensuring the generation of secure and unpredictable sequences (for heightened security needs, consider using SecureRandom). However, there are circumstances, particularly during testing and debugging processes, where manually setting the seed can be beneficial.
This manual seed setting can be accomplished using the ‘srand’ method as follows:
Kernel.srand(1)
By initializing the seed in this manner, the outcome of the random number generator becomes predictable. For instance, using the seed value of 1:
Array.new(5) { rand(1..10) }
# [6, 9, 10, 6, 1]
Reinitializing the generator with the same seed value of 1 will reproduce the same sequence, starting with 6, followed by 9, 10, and so on. This feature is particularly useful for ensuring consistent outcomes in scenarios where repeatability is required.
Conclusion
Ruby’s ability to generate random numbers and strings offers a great deal of flexibility to developers. From gaming simulations to secure token generation, the random generation tools provided by the language can be easily put to excellent use. With their thorough understanding, developers can ensure their code is more dynamic, secure, and versatile.