In Ruby programming, lambdas, procs, and blocks are fundamental elements that significantly influence the functionality and organization of scripts. However, for many developers new to Ruby, understanding these concepts can be initially challenging. This guide is designed to demystify these elements, explaining their definitions and operational principles. By delving into their intricacies, we aim to provide clarity and insight into how they contribute to program dynamics. Through this journey, you’ll gain valuable knowledge that will greatly improve your proficiency in Ruby coding.
A Practical Guide to Ruby Blocks
Ruby’s blocks serve as concise, anonymous functions capable of being passed into methods seamlessly. Enclosed within either a do/end statement or curly brackets {}, blocks offer versatility and can accommodate multiple arguments. Argument names are delineated between two pipe | characters for clarity. If you’ve ever utilized the ‘each’ method in Ruby, you’ve already interfaced with blocks! Below is a simple example to illustrate their usage:
Here’s an illustration:
# Form 1: recommended for single line blocks
[1, 2, 3].each { |num| puts num }
^^^^^ ^^^^^^^^
block block
arguments body
# Form 2: recommended for multi-line blocks
[1, 2, 3].each do |num|
puts num
end
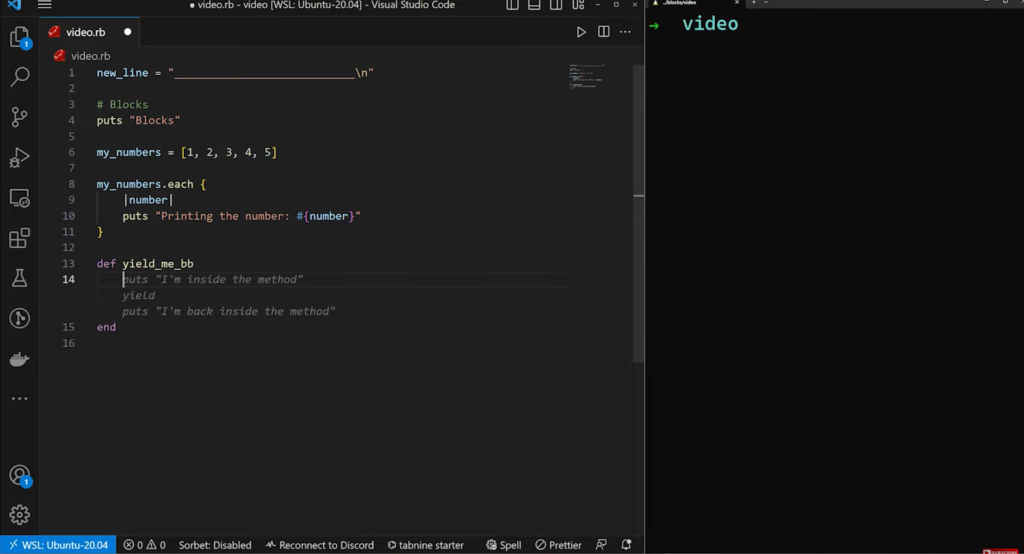
In Ruby, blocks offer a convenient way to encapsulate logic for later use. This could involve tasks like writing data to a file, comparing elements, or displaying error messages.
Understanding the Ruby Yield Keyword
What exactly does the ‘yield’ keyword signify in Ruby? In Ruby, ‘yield’ serves as a fundamental keyword that invokes a block whenever it’s utilized within a method. Essentially, it’s the mechanism by which methods make use of blocks. When you employ the ‘yield’ keyword, the code enclosed within the associated block is executed, carrying out its intended tasks. This process mirrors the invocation of a standard Ruby method. To better grasp this concept, consider the following example:
def print_once
yield
end
print_once { puts "Block is being run" }
When you call the function print_once and pass a block to it, the content within that block is executed. Consequently, the message ‘Block is being run’ will be displayed on the screen.
Fun fact: Did you know that the yield keyword can be used multiple times within a method? Each time you invoke yield, the block associated with it will execute. This behavior is akin to repeatedly calling the same method.
For instance:
def print_twice
yield
yield
end
print_twice { puts "Hello" }
# "Hello"
# "Hello"
Similarly to methods, you have the flexibility to pass any number of arguments to yield.
Example:
def one_two_three
yield 1
yield 2
yield 3
end
one_two_three { |number| puts number * 10 }
# 10, 20, 30
In this scenario, these arguments become the parameters of the block.
For instance, in this example, it’s ‘number’.
Named and Unnamed Code Blocks in Programming
In programming, code blocks can be categorized into two types: “named” and “unnamed”.
A “named” block is one where you assign a name within the method’s parameters. This allows you to pass the block to other methods or store it in a variable for future use.
For instance, consider the following code:
def explicit_block(&block)
block.call # same as yield
end
explicit_block { puts "Explicit block called" }
Pay attention to the &block parameter...
This is how you designate the block's identifier!
Verifying Code Block Presence in Method Calls: Techniques
Attempting to execute a yield command without an accompanying block results in a “no block given (yield)” error. This common issue arises when a method designed to interact with a block is invoked without one.
To circumvent this problem and ensure robust code execution, the block_given? method serves as an essential tool. It allows for the verification of whether a block has been provided alongside the method call.
Here's how you can apply it:
def do_something_with_block
return "No block given" unless block_given?
yield
end
Incorporating this check effectively safeguards against potential errors, ensuring that your method behaves gracefully when invoked without an expected block.
Lambda Expressions: Definition and Utilization
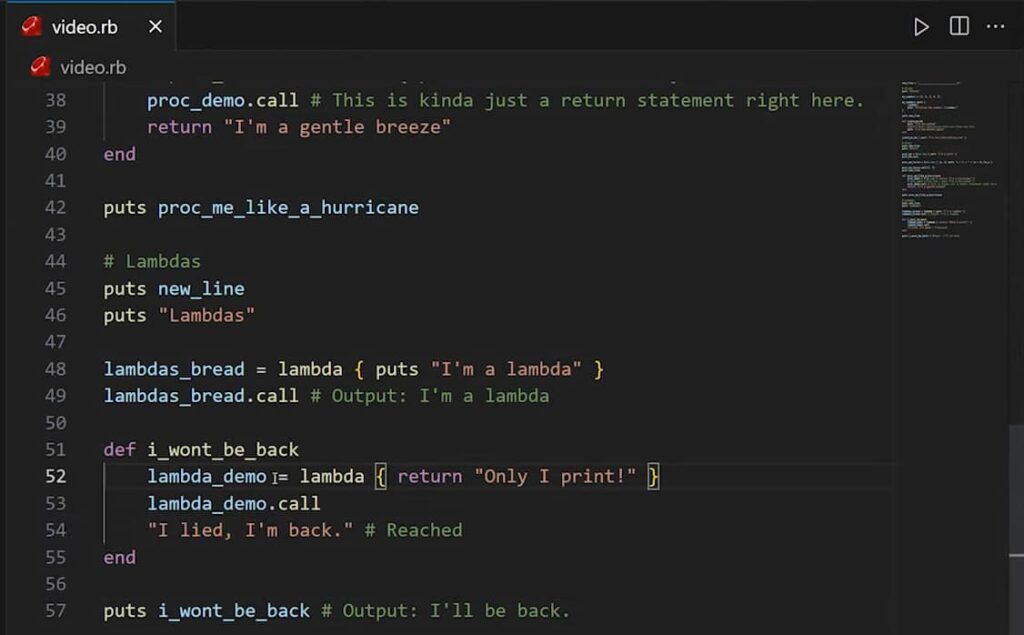
Lambda expressions, a fundamental concept in programming, enable the creation of anonymous functions using a concise syntax. These expressions, often referred to as “lambdas,” can be assigned to variables, allowing for their reuse throughout code.
In Ruby, the essence of defining a lambda is captured through a specific syntax. For instance, a lambda that outputs a message can be defined and invoked as follows:
say_something = -> { puts "This is a lambda" }
Alternatively, Ruby allows the use of the word lambda for defining a lambda, offering syntactic variety. Regardless of the syntax used, creating a lambda does not execute its code immediately. Execution requires explicitly calling the lambda, similar to how methods are invoked upon calling.
Here’s an illustrative example:
Example:
say_something = -> { puts "This is a lambda" }
say_something.call
# "This is a lambda"
Other methods exist for calling a lambda function, and knowing about them is helpful. However, for clarity’s sake, sticking with the ‘call’ method is recommended.
Here’s the list:
my_lambda = -> { puts "Lambda called" }
my_lambda.call
my_lambda.()
my_lambda[]
my_lambda.===
Arguments can also be passed to lambdas. Here's an example:
times_two = ->(x) { x * 2 }
times_two.call(10)
# 20
Passing an incorrect number of arguments to a lambda will result in an exception being raised, similar to a regular method.
Comparing Lambdas and Procs
Procs share a very similar concept with lambdas, but there are distinctions between the two, particularly in their creation.
Example:
my_proc = Proc.new { |x| puts x }
There isn’t a separate Lambda class; instead, a lambda is essentially a specialized Proc object. If you examine the instance methods available in the Proc class, you’ll find a method called lambda?.
Now, when it comes to arguments, a proc behaves differently from a lambda:
t = Proc.new { |x,y| puts "I don't care about arguments!" }
t.call
# "I don't care about arguments!"
Another point of divergence between procs and lambdas lies in their response to a return statement.
A lambda will execute a normal return, similar to a regular method.
Conversely, a proc will attempt to return from the current context.
Here’s what this distinction entails:
When you execute the following code, you’ll observe that the proc triggers a LocalJumpError exception.
This occurs because you cannot return from the top-level context.
Try the following:
# Should work
my_lambda = -> { return 1 }
puts "Lambda result: #{my_lambda.call}"
# Should raise exception
my_proc = Proc.new { return 1 }
puts "Proc result: #{my_proc.call}"
If the proc were contained within a method, invoking return would equate to returning from that method.
This is exemplified in the following demonstration.
def call_proc
puts "Before proc"
my_proc = Proc.new { return 2 }
my_proc.call
puts "After proc"
end
p call_proc
# Prints "Before proc" but not "After proc"
Here’s a summary highlighting the distinctions between procs and lambdas:
- Lambdas are defined using -> {} notation, while procs use Proc.new {};
- Procs return from the current method context, whereas lambdas return from the lambda itself;
- Procs do not enforce the correct number of arguments, while lambdas will raise an ArgumentError exception if the number of arguments is incorrect;
- Analyzing this list, it’s evident that lambdas closely resemble regular methods compared to procs.
Understanding Closures in Ruby
In Ruby, both procs and lambdas possess an additional noteworthy characteristic: they capture the current execution scope.
This concept, often referred to as closure, entails that a proc or lambda retains values such as local variables and methods from the context in which it was defined.
These closures do not store the actual values but rather a reference to them. Consequently, if the variables undergo changes after the proc or lambda is created, it will always reflect the latest version.
Let’s illustrate this with an example:
def call_proc(my_proc)
count = 500
my_proc.call
end
count = 1
my_proc = Proc.new { puts count }
p call_proc(my_proc) # What does this print?
In this illustration, a local variable named count is initialized with a value of 1. Additionally, there’s a proc named my_proc, and a method call_proc designed to execute (via the call method) any proc or lambda provided as an argument.
What output do you anticipate from this program?
At first glance, one might expect the program to output 500, but due to the ‘closure’ effect, it will actually print 1.
This occurs because the proc references the value of count from the context in which it was defined, which is outside the method definition.
Understanding the Binding Class in Ruby
Where exactly do Ruby procs and lambdas store this scope information?
Let’s delve into the Binding class. When you generate a Binding object using the binding method, you establish an ‘anchor’ to the specific point in the code.
Every variable, method, and class defined at this juncture becomes accessible later through this object, irrespective of the scope you’re in at that moment.
Example:
def return_binding
foo = 100
binding
end
# Foo is available thanks to the binding,
# even though we are outside of the method
# where it was defined.
puts return_binding.class
puts return_binding.eval('foo')
# If you try to print foo directly you will get an error.
# The reason is that foo was never defined outside of the method.
puts foo
Put simply, executing code within the context of a binding object is akin to having that code placed at the precise location where the binding was defined, reminiscent of the ‘anchor’ metaphor.
While using binding objects directly may not always be necessary, it’s beneficial to be aware of their functionality.
Conclusion
Throughout this discussion, you gained insights into the functioning of blocks, grasped distinctions between Ruby procs and lambdas, and delved into the concept of the “closure” effect inherent in block creation. One aspect left unexplored is the curry method. This method enables the partial or complete passing of required arguments. When only a subset of arguments is provided, a new proc is generated with these arguments pre-loaded. Upon supplying all required arguments, the proc is executed accordingly.