In the realm of Ruby programming, the concept of time finds its embodiment in the form of a class aptly named ‘Time’. This class serves as a conduit through which one can precisely encapsulate a particular juncture in the continuum. Delve into the depths of this article, and you shall unlock the secrets requisite to ascend to the coveted status of a Ruby time sorcerer!
Exploring the Versatility of Ruby’s Time Class
In the realm of Ruby programming, the Time class serves as a versatile tool, empowering developers to handle dates and times efficiently within their applications. Understanding the fundamentals of this class not only enables you to manipulate temporal data but also opens up a realm of possibilities for building dynamic and time-sensitive functionalities.
Breaking Down the Components:
When dealing with time and date information in Ruby, it’s crucial to grasp the key components that the Time class encapsulates:
- Date Components:
- Day;
- Month;
- Year.
- Time Components:
- Hours;
- Minutes;
- Seconds.
These components form the backbone of temporal representation within the Time class, allowing precise tracking and manipulation of temporal data.
Storing Temporal Information:
Behind the scenes, the Time class stores temporal information as the number of seconds since the Epoch, commonly referred to as Unix time. This standardized approach ensures consistency and interoperability across various systems and platforms.
- Initialization Options: Initiating a Time object can be achieved through several methods, offering flexibility to accommodate diverse programming needs;
- Using Current Time: Utilize Time.now to obtain an object representing the current time;
- Unix Timestamp: Employ the Time.at method in conjunction with a Unix timestamp to create a Time object;
- Specifying Date and Time: Leverage Time.new by providing numerical values representing the year, month, day, hour, minute, and second.
Here’s a glimpse of these initialization methods in action:
Time.now
# 2024-02-26 11:30:15 +0000
Time.new(2024, 2, 26)
# 2024-02-26 00:00:00 +0000
Time.at(1645870215)
# 2024-02-26 11:30:15 +0000
Interrogating Time Objects:
Once a Time object is instantiated, you can delve into its temporal intricacies by querying various attributes:
Day, Month, and Hour: Obtain specific details about the day, month, and hour represented by a Time object.
t = Time.now
puts t.day
puts t.month
puts t.hour
Day of the Week: Determine whether a given date corresponds to a particular day of the week using predicate methods.
t = Time.now
puts t.monday?
puts t.sunday?
puts t.friday?
Exploring Time Zones in Ruby
Understanding time zones in Ruby is essential for accurate time management and synchronization across different locations. When working with Time objects, each instance is associated with a specific time zone, providing crucial information for various applications. Let’s delve deeper into how Ruby handles time zones and how you can effectively utilize this feature:
Accessing Time Zone Information
Utilize the zone method: With this method, you can easily retrieve the current time zone abbreviation associated with a Time object. It offers quick insights into the time zone setting, aiding in time-related computations and adjustments.
Obtaining time zone offset: The utc_offset method allows you to retrieve the time zone offset in seconds. By dividing the result by 3600, you can convert it into hours, providing a clearer understanding of the temporal disparity between different regions.
Example:
t = Time.now
puts t.zone
# Output: "CET"
puts t.utc_offset / 3600
# Output: 1
Exploring UTC: Ruby facilitates accessing Coordinated Universal Time (UTC) effortlessly. By invoking utc on a Time object, you can obtain the current time in UTC, ensuring standardized time representation across diverse environments.
Enhancing Time Formatting with Ruby
Customizing the format of Time objects in Ruby enables developers to tailor temporal representations according to specific requirements. The strftime method serves as a powerful tool for achieving this customization, accommodating a myriad of formatting options. Let’s uncover the versatility of strftime and its practical applications:
Usage of strftime
- Understanding format specifiers: strftime relies on format specifiers embedded within a string to generate formatted time representations. These specifiers dynamically substitute with corresponding time values, offering granular control over the output format;
- Mimicking printf behavior: If you’re familiar with the printf method, leveraging strftime will feel intuitive. Both methods share the concept of utilizing placeholders to structure output, facilitating seamless transition for developers accustomed to C-style formatting.
Examples:
time = Time.new
puts time.strftime("%d/%m/%Y") # Output: "05/12/2015"
puts time.strftime("%k:%M") # Output: "17:48"
puts time.strftime("%I:%M %p") # Output: "11:04 PM"
puts time.strftime("Today is %A") # Output: "Today is Sunday"
puts time.strftime("%d of %B, %Y") # Output: "21 of December, 2015"
puts time.strftime("Unix time is %s") # Output: "Unix time is 1449336630"
Flexible formatting options: strftime empowers developers to craft diverse time representations. Whether you require a concise time display or a detailed date format, the method accommodates various formatting needs with ease, fostering enhanced user experience and data presentation.
Understanding strftime Format Codes: A Comprehensive Guide
FORMAT | DESCRIPTION |
---|---|
%d | Day of the month (01..31) |
%m | Month of the year (01..12) Use %-m for (1..12) |
%k | Hour (0..23) |
%M | Minutes |
%S | Seconds (00..60) |
%I | Hour (1..12) |
%p | AM/PM |
%Y | Year |
%A | Day of the week (name) |
%B | Month (name) |
Generating a Timestamp
Timestamps are invaluable for marking specific moments in time, independent of any particular time zone. Whether you’re tracking events, managing data, or debugging code, generating accurate timestamps is a fundamental skill.
How to Generate a Timestamp:
Using Ruby’s Time.now.to_i Function: This command returns the current time as a timestamp, represented by the number of seconds elapsed since the Unix epoch (January 1, 1970, 00:00:00 UTC).
Time.now.to_i
# Example output: 1645907658
- Understanding the UTC (Coordinated Universal Time): The timestamp obtained is in UTC, ensuring consistency across different time zones;
- Applications: Timestamps find utility in various scenarios, including logging events, synchronizing data, and measuring performance.
Time Difference (Addition & Subtraction)
Manipulating time objects allows for dynamic adjustments, whether projecting future events or reflecting on past occurrences.
- Adding Time:
Time Object Addition: By adding seconds to the current time, you can project events into the future.
# Add ten seconds
future_time = Time.new + 10
Verifying Time Passage: Utilize comparison operators to check if a particular time has elapsed.
Time.new > future_time
- Subtracting Time:
Calculating Time Intervals: Understanding time representations in seconds facilitates precise adjustments. For instance, a day consists of:
60 seconds * 60 minutes * 24 hours = 86,400 seconds.
Subtracting Time Intervals:
# Subtracting a day from the current time
past_time = Time.now - 86400
- Leveraging Rails:
In Rails applications, time manipulations are simplified through convenient methods like 1.day, providing intuitive syntax for subtracting time intervals.
# Subtracting a day in Rails
Time.now - 1.day
Understanding the Date Class
The Date class is a fundamental component in Ruby programming, serving as a cornerstone for managing dates within your applications. Unlike its companion, the Time class, Date operates on a higher abstraction level, focusing solely on dates rather than incorporating the concept of time, including hours, minutes, and seconds. Here’s what you need to know to harness its power effectively:
- Internal Representation: Dates in the Date class are stored purely in terms of days, devoid of any time-related attributes. This simplicity streamlines operations concerning dates and facilitates cleaner code;
- Getting Started: To utilize the Date class in your Ruby scripts, ensure you’ve required the ‘date’ module. Once integrated, accessing the current date is a breeze with the Date.today method;
- Differentiation from Time: Unlike Time, where Time.new fetches the current time, in Date, Date.new doesn’t represent today’s date but instead returns a negative date. Understanding this distinction is vital to avoid unintended errors in your code;
- Date Arithmetic: Manipulating dates with the Date class involves adding or subtracting days rather than seconds. This aligns with the class’s focus on dates alone and simplifies date-related calculations.
Usage Examples:
# Examples of Date usage
Date.today # Fetches the current date
Date.new # Returns a negative date
Date.today + 1 # Adds one day to the current date
Considerations: Due to its limitation to dates only, Date class should be employed when time-related precision is unnecessary. If your application necessitates tracking time alongside dates, the Time class might be more appropriate.
Date & Time Parsing Techniques
Parsing dates and times accurately from user inputs or external sources is crucial for robust application functionality. Ruby offers several methods for parsing dates, each tailored to specific requirements. Let’s delve into these techniques:
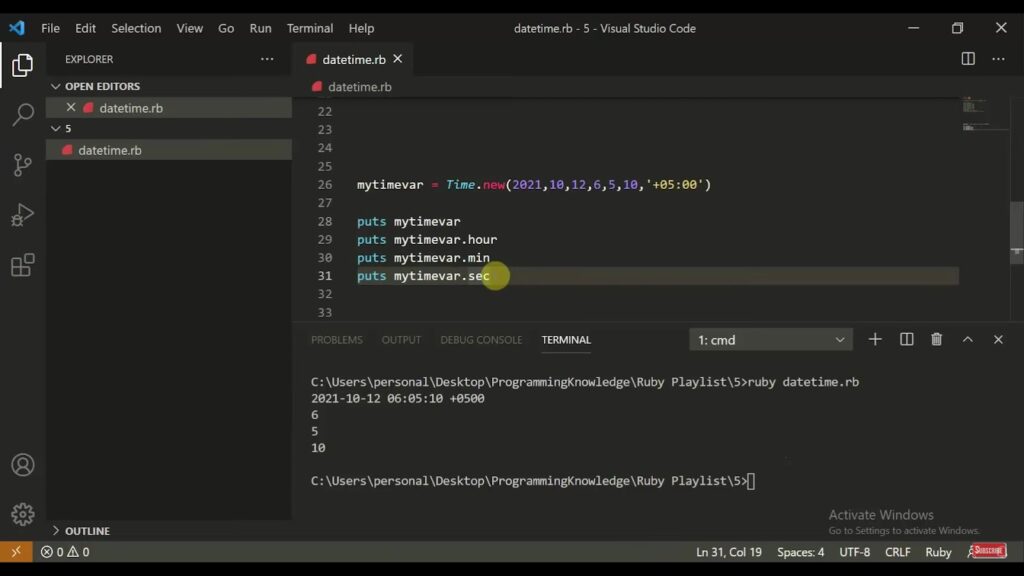
- Date.parse: This versatile method attempts to interpret any string resembling a date, employing a heuristic algorithm to discern the input format. While convenient, its heuristic nature may yield unexpected outcomes in certain cases;
- Date.iso8601: For stricter parsing adhering to the ISO 8601 standard, Date.iso8601 provides a reliable solution. This method expects dates in the format of ‘year-month-day’, ensuring consistent and unambiguous parsing;
- Custom Parsing with Date.strptime: When precise control over input format is paramount, Date.strptime shines. Leveraging format specifiers akin to strftime, this method empowers developers to define custom parsing rules tailored to specific date formats;
- Parsing Time with Time.parse: While Date handles dates exclusively, parsing time necessitates the use of the Time class. Time.parse seamlessly converts strings representing time into Time objects, simplifying time-related operations.
Usage Examples:
# Examples of Date and Time parsing
Date.parse("10/10/2010") # -> 2010-10-10
Date.iso8601("2018-10-01")
Date.strptime("3 of September", "%d of %B") # 2015-09-03
Time.parse("September 20 18:00")
Time.strptime("1 of December 2017", "%d of %B %Y")
Differentiating Date.parse and Time.parse: While both Date.parse and Time.parse serve parsing purposes, the former yields Date objects, focusing solely on dates, while the latter returns Time objects, encompassing both date and time components.
Exploring Date Constants
Understanding the Date class in Ruby unveils a treasure trove of constants, providing convenience and efficiency in date manipulations. Delve into these date constants to harness their power:
Month and Weekday Arrays: The Date class offers arrays that encapsulate the months of the year and the days of the week, enhancing your ability to work with dates effortlessly.
Access month names directly mapped to their respective numbers, starting from index 1.
Seamlessly manipulate weekdays starting from Sunday, or customize to begin from Monday using the rotate method.
# Example Usage:
Date::MONTHNAMES # (index 0 = nil)
Date::DAYNAMES.rotate(1)
Enhanced Mapping: Gain insights into month-to-number and weekday-to-name mappings, facilitating precise date operations.
Leverage direct access to month numbers and names for seamless navigation.
Customize the starting day of the week to align with specific requirements, enhancing flexibility in date handling.
# Example:
Month_Number_Mapping = {
1 => "January",
2 => "February",
# Add more mappings...
}
Weekday_Name_Mapping = {
0 => "Monday",
1 => "Tuesday",
# Add more mappings...
}
Optimized Date Management: Empower your code with intuitive date manipulation, optimizing efficiency and precision.
Utilize predefined arrays to streamline month and weekday operations, reducing complexity.
Seamlessly integrate customizations to align with diverse project requirements, ensuring compatibility and ease of use.
Unveiling the DateTime Class
Dive into the intricacies of the DateTime class, a dynamic extension of the Date class, unlocking unparalleled versatility in date and time management:
Extended Functionality: Experience a seamless transition from dates to precise timestamps, expanding the horizons of your applications.
Embrace the DateTime class as an extension of the Date class, enriching your code with advanced temporal capabilities. Store precise timestamps with seconds granularity, enabling fine-grained temporal calculations and representations.
# Example Usage:
require 'date'
DateTime.superclass
DateTime.now
Performance Considerations: Navigate the trade-offs between Time and DateTime, optimizing performance without compromising functionality.
Assess the performance discrepancies between Time and DateTime, considering factors like implementation language and execution speed. Opt for Time for enhanced performance, leveraging its C implementation to accelerate time-related operations.
# Performance Comparison:
Time: 2644596.6 i/s
DateTime: 231634.8 i/s - 11.42x slower
Strategic Implementation: Strategically leverage Time or DateTime based on your project’s performance and functionality requirements.
- Choose Time for computationally intensive tasks demanding high-speed execution, prioritizing efficiency;
- Embrace DateTime for nuanced temporal manipulations requiring precision and granularity, balancing performance with functionality.
Mastering ActiveSupport – Time & Date Methods
Embark on a journey through ActiveSupport, unraveling a plethora of time and date methods tailored for Rails enthusiasts:
Enhanced Functionality: Explore ActiveSupport’s arsenal of time and date methods, elevating your Rails experience with unparalleled convenience.
Unleash the power of Rails-specific methods like 3.days.ago for intuitive date manipulations, exclusive to ActiveSupport. Embrace a seamless transition between durations, timestamps, and date calculations, augmenting productivity and code readability.
# Example Usage:
1.hour.to_i # 3600
1.day # ActiveSupport::Duration
3.days.ago # ActiveSupport::TimeWithZone
Effortless Time Arithmetic: Dive into time arithmetic with ActiveSupport, simplifying complex temporal calculations with ease.
Perform time-based arithmetic effortlessly, leveraging ActiveSupport’s intuitive syntax for precise date calculations. Seamlessly navigate time zones and daylight saving adjustments, ensuring accurate temporal representations in diverse environments.
# Example Usage:
Time.now + 1.day
Tailored Date Formatting: Elevate your application’s user experience with customized date formatting options, enhancing readability and aesthetics.
Craft visually appealing date formats using ActiveSupport’s versatile formatting methods, tailored to your application’s design language. Seamlessly integrate date formatting into your codebase, enhancing user interaction and interface aesthetics.
# Example Usage:
date = Date.today
date.to_formatted_s(:short) # "16 Jul"
date.to_formatted_s(:long) # "July 16, 2018"
Conclusion
In conclusion, by delving into the intricacies of Ruby’s Time class, you have unearthed the means to wield temporal power with finesse. Armed with this knowledge, you are poised to navigate the vast landscape of time manipulation within Ruby programming, transcending from mere practitioner to adept. Embrace your newfound understanding and continue to explore the realms of Ruby programming with confidence and curiosity. The journey to mastery is perpetual, and with each revelation, you inch closer to the zenith of proficiency as a Ruby time wizard.