Redis is a powerful in-memory database that offers various data structures for efficient storage and retrieval of data. Unlike traditional SQL databases, Redis is designed to be fast and lightweight, making it a popular choice for developers working with large datasets. In this article, we will explore how to use Redis in your Ruby applications, covering everything from installation to advanced features.
Installing Redis Locally
Before we dive into using Redis in Ruby, we first need to install the Redis server on our local machine. This process will vary depending on your operating system, but the basic steps are the same. If you’re using Ubuntu, you can use apt to install the Redis server. On Mac, you can use brew, and on Windows 10, you’ll need to set up the Windows Subsystem for Linux (WSL). Once the server is installed, you can start it by running redis-server in your terminal.
To connect to the server, you can use the redis-cli command. However, if you want to work with Redis in your Ruby applications, it’s recommended to use a gem instead. There are several gems available for interacting with Redis, such as oxblood, redic, and redis-rb. However, the official recommendation from the Redis developers is to use the redis-rb gem. You can install it by running gem install redis in your terminal.
Using Redis Gems
Now that we have the redis-rb gem installed, we can start working with Redis in our Ruby applications. The first step is to establish a connection to the Redis server using the Redis.new method. This will return an instance of the Redis class, which we can use to interact with the database.
Adding Data to Redis
Let’s start by adding some data to our Redis database. We can do this using the set method, which takes in a key and a value as parameters. For example, we can add a key called a with a value of 1 by running the following code:
redis = Redis.new
redis.set("a", 1)
We can then retrieve the value of a by using the get method:
redis.get("a")
# => "1"
Working With Data Structures
One of the main advantages of using Redis is its support for various data structures. Let’s take a look at some of the most commonly used ones.
Key/Value Storage
As we saw in the previous example, Redis allows us to store data using a key/value pair. This makes it easy to retrieve data quickly, as long as we know the key associated with it. We can also use the mset method to set multiple key/value pairs at once:
redis.mset("b", 2, "c", 3)
Lists
Redis also offers support for lists, which are similar to arrays in Ruby. We can use the lpushand rpush methods to add items to the beginning and end of a list, respectively. We can also use the lrange method to retrieve a range of items from a list:
redis.lpush("list", "item1")
redis.rpush("list", "item2")
redis.lrange("list", 0, -1)
# => ["item1", "item2"]
Sets
Sets in Redis are unordered collections of unique values. We can use the sadd method to add items to a set, and the smembers method to retrieve all the items in a set:
redis.sadd("set", "item1")
redis.sadd("set", "item2")
redis.smembers("set")
# => ["item1", "item2"]
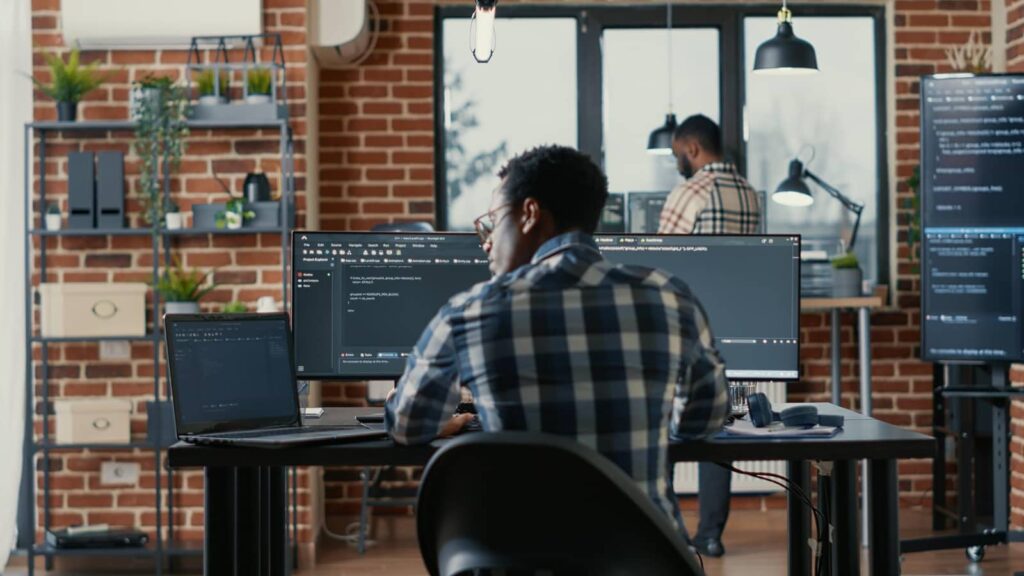
Working With The Documentation
As with any new technology, it’s important to familiarize yourself with the documentation before diving into using Redis in your Ruby applications. The official Redis website offers comprehensive documentation and guides on how to use the database effectively. It’s also worth checking out the redis-rb gem’s documentation for specific examples and usage instructions.
One important aspect to keep in mind when working with Redis is its command syntax. Redis commands follow a specific pattern, which consists of a command name followed by its arguments. For example, the get command we used earlier follows this pattern: get key. This makes it easy to understand and remember the various commands available in Redis.
How to Use Sorted Sets in Redis
Sorted sets are a unique data structure offered by Redis that combines the features of a set and a sorted list. Each item in a sorted set is associated with a score, which is used to determine the order of the items in the set. This allows us to retrieve items from the set in a specific order, making it useful for tasks such as leaderboards or rankings.
To add an item to a sorted set, we can use the zadd method, which takes in a score and a value as parameters. We can then retrieve the items in the set using the zrange method, which returns a range of items based on their scores:
redis.zadd("leaderboard", 100, "player1")
redis.zadd("leaderboard", 200, "player2")
redis.zrange("leaderboard", 0, -1)
# => ["player1", "player2"]
Understanding Keys, Values & Namespacing
In Redis, keys are used to identify and retrieve data from the database. It’s important to choose meaningful and unique keys for your data to avoid conflicts. Additionally, Redis allows us to group related keys together using a technique called namespacing.
Namespacing involves adding a prefix to all your keys to indicate their purpose or origin. This can be useful when working with multiple applications that use the same Redis server. We can set a namespace by using the namespace method on our Redis instance:
redis = Redis.new
redis.namespace = "myapp"
This will add the prefix myapp: to all our keys, making it easier to differentiate them from other keys in the database.
Data Persistence in Redis
One of the main differences between Redis and traditional SQL databases is its approach to data persistence. By default, Redis does not persist data to disk, meaning that all data will be lost if the server is restarted. However, we can enable persistence by using the saveor appendonly options in the Redis configuration file.
The save option allows us to specify how often the database should be saved to disk, while the appendonly option enables an append-only file that logs every write operation to the database. Both of these options come with their own trade-offs, so it’s important to understand the implications before enabling them.
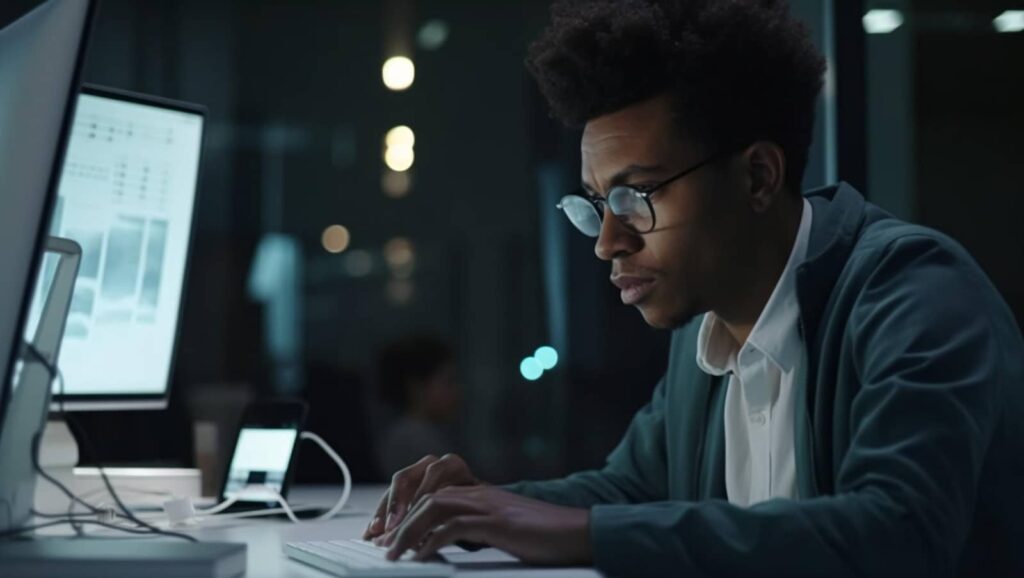
Using Redis as a Rails Cache
If you’re working with Ruby on Rails, you can easily integrate Redis as a cache store for your application. Caching is a common practice in web development, where frequently accessed data is stored in memory to improve performance. Redis is an excellent choice for caching due to its fast read and write speeds.
To use Redis as a cache store in Rails, we first need to install the redis-rails gem. We can then configure our application to use Redis as the default cache store by adding the following line to our config/application.rb file:
config.cache_store = :redis_cache_store, { url: "redis://localhost:6379/0" }
This will use the default Redis configuration, but we can also specify a custom configuration if needed. We can then use the Rails.cache object to interact with the cache store in our application.
Redis F.A.Q
Yes, Redis is designed to handle large datasets efficiently. Its in-memory storage and support for various data structures make it a popular choice for working with large amounts of data.
Yes, Redis has official clients available for various programming languages, including Java, Python, and Node.js. This makes it easy to integrate Redis into applications written in different languages.
Yes, Redis supports transactions through the use of the MULTI and EXEC commands. These allow us to group multiple operations together and execute them as a single unit.
Summary
In this article, we explored how to use the Redis database in Ruby. We started by installing Redis locally and using the redis-rb gem to interact with the database. We then looked at some of the most commonly used data structures in Redis, such as key/value storage, lists, and sets. We also discussed the importance of understanding the documentation and how to use sorted sets in Redis.
We then delved into topics such as keys, values, and namespacing, as well as data persistence in Redis. Finally, we saw how we can use Redis as a cache store in Ruby on Rails applications. With its fast read and write speeds, support for various data structures, and easy integration with other programming languages, Redis is a powerful tool for managing large datasets.