Have you ever wondered how computers make decisions? How do they know what to do in different situations? Well, in the world of programming, this is done through conditional statements. And in the world of Ruby, this is done through if and else statements. In this article, we will dive deep into the world of Ruby if and else statements, learn about different types of conditions, and explore some special symbols that can be used in strings. So let’s get started!
How Do You Make Decisions in Ruby?
In simple terms, making decisions in Ruby means using conditional statements to perform certain actions based on specific conditions. These conditions can be anything from checking the value of a variable to comparing two values. Let’s take a look at some examples:
- If the room is too cold, turn on the heater;
- If we don’t have enough stock of a product, then send an order to buy more;
- If a customer has been with us for more than 3 years, then send them a thank you gift.
These are just a few examples of how we can use conditional statements to make decisions in Ruby. Now, let’s see how we can write these decisions in code using if statements.
Types Of Conditions
In Ruby, there are various types of conditions that we can use in our if statements. These conditions are represented by different symbols, each with its own meaning. Here’s a table that summarizes these symbols and their meanings:
Symbol | Meaning |
---|---|
< | Less than |
> | Greater than |
== | Equals |
!= | Not equals |
>= | Greater or equal to |
<= | Less or equal to |
One important thing to note here is that we use two equal signs (==) to check for equality, not just one. This is because a single equal sign (=) is used for assignment in Ruby. So if we want to check if two values are equal, we need to use two equal signs.
Ruby Unless Statement
Apart from the if statement, Ruby also has an unless statement that can be used to make decisions. The unless statement is the opposite of the if statement, meaning it will only execute the code inside its condition if the condition is false. Let’s take a look at an example:
stock = 10
unless stock > 0
puts "Sorry, we are out of stock!"
end
In this example, the code inside the unless statement will only be executed if the value of stock is not greater than 0, which means the stock is equal to or less than 0. So in this case, the message “Sorry, we are out of stock!” will be printed.
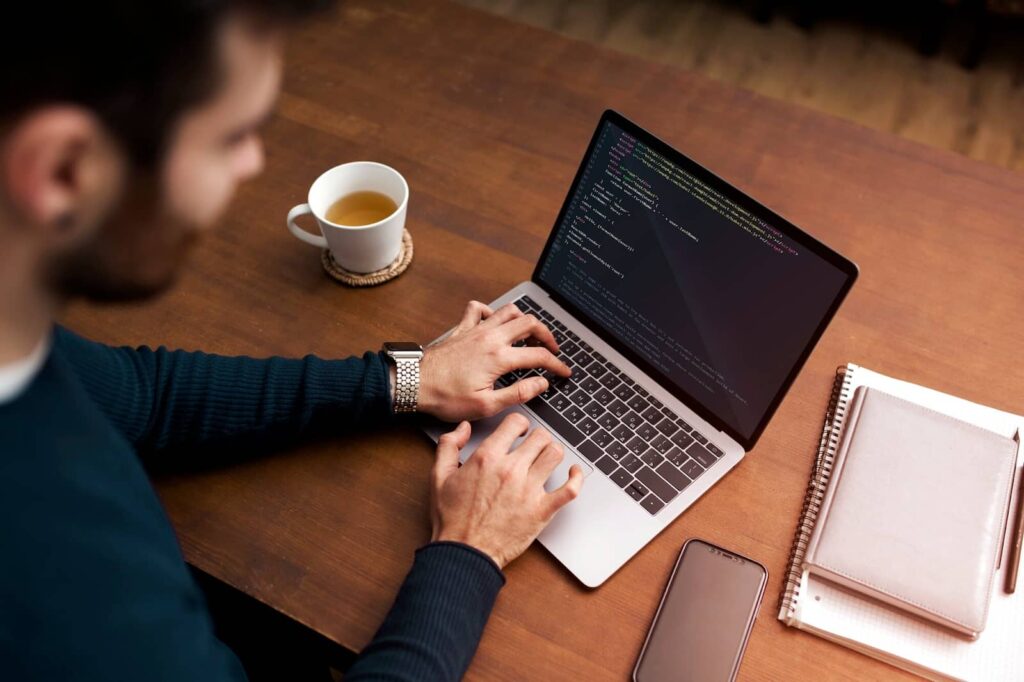
The If Else Statement
Now, let’s move on to the if else statement. This statement allows us to specify what should happen if the condition is true and what should happen if the condition is false. Here’s an example:
age = 25
if age >= 18
puts "You are old enough to vote."
else
puts "You are not old enough to vote."
end
In this example, if the value of age is greater than or equal to 18, the first message will be printed. Otherwise, the second message will be printed.
How to Use Multiple Conditions
Sometimes, we may need to check multiple conditions before executing a certain piece of code. In such cases, we can use logical operators like && (and), || (or), and ! (not). These operators allow us to combine multiple conditions and create more complex conditions. Let’s take a look at an example:
age = 25
if age >= 18 && age <= 30
puts "You are in the prime of your life."
end
In this example, the code inside the if statement will only be executed if both conditions are true, meaning the value of age is between 18 and 30.
Things to Watch Out For
When using conditional statements in Ruby, there are a few things that we need to watch out for to avoid unexpected results. One common mistake is using a single equal sign (=) instead of two (==) when checking for equality. Another thing to keep in mind is the order of conditions. If we have multiple conditions, the order in which they are written can affect the outcome.
Special Symbols in Strings
Apart from the symbols we use in conditions, there are also some special symbols that we can use in strings to make our code more readable. These symbols are called escape sequences and they start with a backslash (). Here are some commonly used escape sequences:
Escape Sequence | Meaning |
---|---|
\n | New line |
\t | Tab |
\” | Double quote |
\’ | Single quote |
For example, if we want to print a string that contains a double quote, we can use the escape sequence \” to tell Ruby that the double quote is part of the string and not the end of it. Let’s see an example:
puts "She said, \"I love Ruby!\""
This will print: She said, "I love Ruby!"
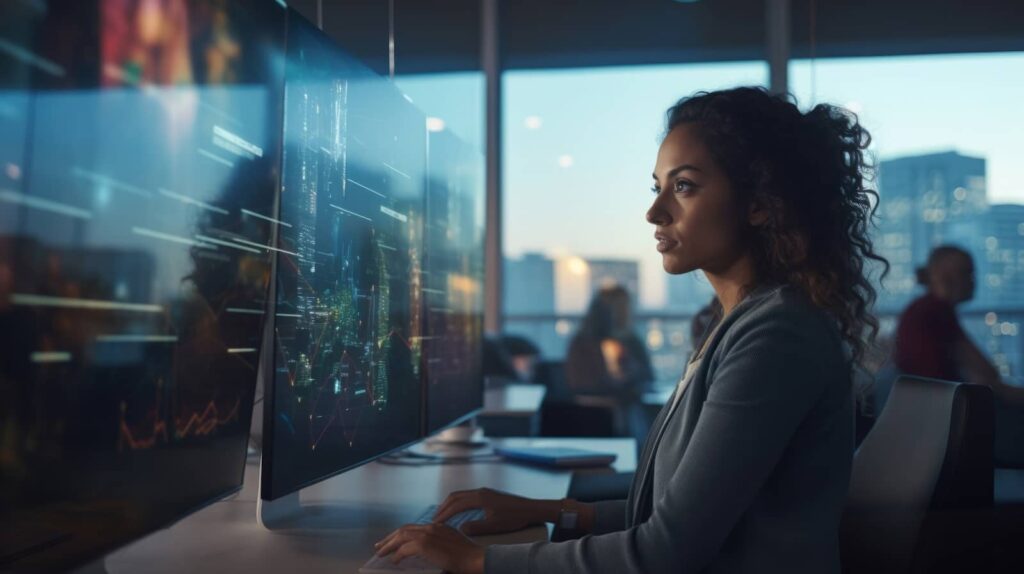
If Construct in One Line
In Ruby, we can also write if statements in one line using the if construct. This is useful when we have a simple condition and don’t want to write multiple lines of code. Here’s an example:
stock = 10
puts "Sorry, we are out of stock!" if stock < 1
This will print the message only if the value of stock is less than 1.
Is There an Alternative?
While if and else statements are commonly used in Ruby, there is an alternative that can be used in some situations. This alternative is called the ternary operator and it allows us to write if statements in a more concise way. Let’s take a look at an example:
age = 25
age >= 18 ? puts("You are old enough to vote.") : puts("You are not old enough to vote.")
This is equivalent to writing:
if age >= 18
puts "You are old enough to vote."
else
puts "You are not old enough to vote."
end
As you can see, the ternary operator can save us some lines of code, but it’s important to use it wisely and only when it makes our code more readable.
Summary
In this article, we learned about conditional statements in Ruby, specifically if and else statements. We explored different types of conditions and how to use them in our code. We also saw some special symbols that can be used in strings and how to write if statements in one line using the if construct. Lastly, we briefly mentioned the ternary operator as an alternative to if and else statements.